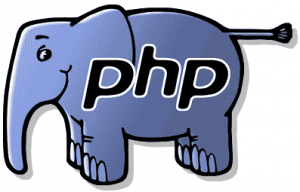
Couple of years ago I wrote an article about two PHP configuration options auto_prepend_file and auto_append_file recently while browsing about PHP minification I got an idea on how to apply this PHP minify code to each and every script executed on the server so that all HTML output from that server are auto minified. This method requires creating a PHP file containing the code to strip whitespaces and newlines before and after the HTML tags and including this file with every PHP script executed on the server using the auto_prepend_file configuration option. For this you need to have access to the php.ini file. This method will work fine even if you are using shared hosting with custom php.ini support.
Create a PHP file outside your document root. If your document root is
/var/www/html/
create the a file named minify.php one level above it
/var/www/minify.php
Copy paste the following PHP code into it
<?php function minify_output($buffer) { $search = array( '/\>[^\S ]+/s', '/[^\S ]+\</s', '/(\s)+/s' ); $replace = array( '>', '<', '\\1' ); if (preg_match("/\<html/i",$buffer) == 1 && preg_match("/\<\/html\>/i",$buffer) == 1) { $buffer = preg_replace($search, $replace, $buffer); } return $buffer; } ob_start("minify_output"); ?>
Save the minify.php file and open the php.ini file. If it is a dedicated server/VPS search for the following option, on shared hosting with custom php.ini add it.
auto_prepend_file = /var/www/minify.php
Save the file and now try accessing any of your PHP scripts, if they output code containing HTML open/close tags (<html>,</html>) you will see the script in action. If you are getting a blank page it means there was an error in the minify.php code, in which case you have to turn on display_errors or check the PHP errorlog.
Note: This solution does NOT minify inline CSS or Javascript.
Working of this code
I got this code from http://www.php.net/manual/en/function.ob-start.php#71953 lets see how it works. The ob_start PHP function turns on output buffering, due to this no output is sent from script except the headers. In this code a function called “minify_output()” is called which takes this “buffer”, minifies it and returns it. Now let us look at each element of the array variable “$search”.
The first element
'/\>[^\S ]+/s'
matches whitespaces after HTML tags including newlines and replaces it with the first element of the “$replace” array variable.
'>'
The second element
'/[^\S ]+\</s'
matches whitespaces and newlines before HTML tags and replaces them with the second element of the “$replace” array variable.
'<'
The third element
'/(\s)+/s'
matches multiple whitespace sequences and removes them.
The “if” loop before the “preg_replace()” function is to ensure minification is performed only if the output is HTML, i.e. only if the output of the PHP script contains the strings “<html” and “</html> will minification be performed. This is to ensure non HTML output is not broken by minifying it. Some PHP scripts are used to output CSS or Javascript dynamically and are called using the <link> or <script> tag
<link rel="stylesheet" type="text/css" href="/styles.php?theme=red"> <script type="text/javascript" src="jscript.php?v=1.2"></script>
In these cases if the output code contained “<“, “>” or multiple whitespace sequences as a part of their CSS or Javascript syntax then minify.php would remove them causing the CSS or Javascript to fail.
Great Solution. Thanks for sharing, Jesin !! But how to remove space between tags without to remove the wordspace in text? This is what we get now (as example):
hmmm, my html example tags with the space between was removed from the form of course, sorry.
Hi Peter,
Please paste the code to Pastbin and share the URL.
I get output of code
Not design
what to do?
Just to put it more clearly, there’s no real need to use the PHP.INI settings at all to use this one nice piece of PHP code. 🙂
You can simply use one-liner right before your website/CMS script main site part starts, to call that minify.php, and of course you can rename it just the way want. The one-liner is: require(‘minify.php’);
In some cases this may also help to solve problems like only-code-output, because, you fine-tune the order of calls.