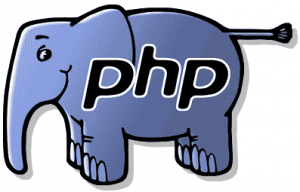
This tutorial explains how to use multiple submit buttons in a HTML form and have PHP handle it. Usually a HTML form has only one submit button but there are situations when you might need to use more than one submit buttons and have PHP check which button has been pressed and an action to be done according to the button pressed. Having multiple submit buttons and handling them through PHP is just a matter of checking the the name of the button with the corresponding value of the button using conditional statements.
In this article I’ll use both elseif ladder and switch case statement in PHP to handle multiple submit buttons in a form.
The HTML part
First go to your HTML form and add as many submit buttons you want inside the <form> tag, with one condition the name attribute of all the submit buttons must be the same like the following
<input type="submit" name="btn_submit" value="Button 1" /> <input type="submit" name="btn_submit" value="Button 2" /> <input type="submit" name="btn_submit" value="Button 3" />
The text entered in the value attribute is shown in the output as the submit button’s caption. Next we’ll move on to the PHP part.
The PHP part
As you might know when the submit button is pressed the browser sends the values of the input elements within that <form> tag to the file specified in the action attribute in the <form> tag. Along with that the “value” of the submit button is also sent, so in the PHP part we’ll check which value is sent using elseif ladder and switch case statements you can use the one you are comfortable with.
<?php if($_REQUEST['btn_submit']=="Button 1") { print "You pressed Button 1"; } else if($_REQUEST['btn_submit']=="Button 2") { print "You pressed Button 2"; } else if($_REQUEST['btn_submit']=="Button 3") { print "You pressed Button 3"; } ?>
Doing the same using switch case
<?php switch ($_REQUEST['btn_submit']) { case "Button 1": print "You pressed Button 1"; break; case "Button 2": print "You pressed Button 2"; break; case "Button 3": print "You pressed Button 3"; break; } ?>
View a demo of using multiple submit buttons in PHP
Thank you very much!
But is this also possible with $_POST instead of $_REQUEST (by the switch method)?