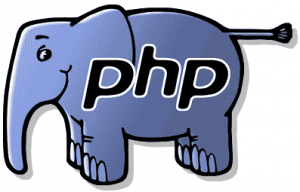
PHP Login page script with three modules, the first one for allowing new users to register, the second for allowing users to login and the third page is a private page which only logged in users can access. Before reading this article make sure you read Connect PHP with MySQL because there is extensive use of PHP mysql functions throughout this article. The registration module uses INSERT query, the login module uses SELECT query to verify whether a user’s credentials exist in the database and creates a session and the private page checks to see if there is a session variable.
Create a database and a table
Create a database which will house the table containing user information such as Full name, User name and password. Execute the following queries to do it
CREATE DATABASE users; CREATE TABLE login (full_name varchar(30) NOT NULL, username varchar(11) NOT NULL, password varchar(30) NOT NULL, PRIMARY KEY(username));
The field username has been set as a primary key so that there are no identical usernames. You can execute this queries either from MySQL command line interface or phpMyAdmin or using the PHP mysql_query() function.
New User Registration Module
This module will allow new users to register themselves on your website. This module will make use of the INSERT query to add user information entered in a HTML form to the database. I’ll explain the PHP part and add a HTML form to it at the end. In he HTML form have text boxes for Full Name, Username, Password, Retype Password. The first objective is to check whether the user has filled in all the text boxes and if its all filled both the passwords should match
$fname=stripslashes(trim($_POST['fname'])); $uname=stripslashes(trim($_POST['uname'])); $pass1=stripslashes(trim($_POST['pass1'])); $pass2=stripslashes(trim($_POST['pass2'])); if(!empty($fname) && !empty($uname) && !empty($pass1) && !empty($pass2)) { if ($pass1==$pass2) { /*Insert values into Database*/ } else { print "Passwords do not match"; } } else { print "Please Fill in all the fields"; }
Now lets come to the interesting part of adding the values into the database. All the PHP mysql functions will come under the nested if loop. So the entire code will be as follows
$fname=stripslashes(trim($_POST['fname'])); $uname=stripslashes(trim($_POST['uname'])); $pass1=stripslashes(trim($_POST['pass1'])); $pass2=stripslashes(trim($_POST['pass2'])); if(!empty($fname) && !empty($uname) && !empty($pass1) && !empty($pass2)) { if ($pass1==$pass2) { mysql_connect("MySQL_Host","Username","Password"); mysql_select_db("users"); $add_user=mysql_query("INSERT INTO login values ('$fname','$uname','$pass1')"); if ($add_user) { print "You've sucessfully registered on our website"; mysql_close(); } } else { print "Passwords do not match"; } } else { print "Please Fill in all the fields"; }
PHP Login Page
The login page will verify whether a particular user exists in the database with the specified username and password. The SELECT query will be used inside the mysql_query() function for this purpose. and if it exists a session will be created and session variables will be initialized. As in the previous module PHP will check if all the fields are filled.
if (!empty($_POST['uname']) && !empty($_POST['pass'])) { $uname=stripslashes(trim($_POST['uname'])); $pass=stripslashes(trim($_POST['pass'])); mysql_connect("MySQL Host","Username","password"); mysql_select_db("users"); $check=mysql_query("SELECT * FROM login WHERE username='$uname' AND password='$pass'"); if(mysql_num_rows($check)!=0) { $details=mysql_fetch_array($check); $_SESSION['display_name']=$details[0]; $_SESSION['username']=$details[1]; $_SESSION['password']=$details[2]; print "Successfully Logged in"; } else { print "Invalid Username/Password"; } } else { print "All fields must be filled"; }
The mysql_num_rows() returns the number of rows from the query executed. So if the user doesn’t exist or if the username/password is invalid an error message is displayed. If they are correct the full_name, username and password are stored as an array in the variable $details which is assigned to three session variables which are created if the credentials are correct. Now it isn’t over after logging in you’ll have to redirect the user to a private area and also if the user accesses this page after logging in it displays the message “All fields must be filled” because the POST variables are empty this time so if you access this after logging in it should redirect you to the private page. Lets do this by adding an if loop on the top which checks if the necessary session variables are set, if they are it will redirect the user to private.php
session_start(); if(isset($_SESSION['username']) && isset($_SESSION['password'])) { header("Location: private.php"); } if (!empty($_POST['uname']) && !empty($_POST['pass'])) { $uname=stripslashes(trim($_POST['uname'])); $pass=stripslashes(trim($_POST['pass'])); mysql_connect("MySQL Host","Username","password"); mysql_select_db("users"); $check=mysql_query("SELECT * FROM login WHERE username='$uname' AND password='$pass'"); if(mysql_num_rows($check)!=0) { $details=mysql_fetch_array($check); $_SESSION['display_name']=$details[0]; $_SESSION['username']=$details[1]; $_SESSION['password']=$details[2]; header("Location: private.php"); } else { print "Invalid Username/Password"; } } else { print "All fields must be filled"; }
The final touch in the login.php page is to add the HTML form to it. Of course you can also store it in a separate HTML file but it will be less professional. Adding the HTML code along with the PHP code is simple. The whole code is placed inside an if else loop which checks if the $_POST variables are set if they are it proceeds with the other operations else the HTML login form is shown
<?php session_start(); if(isset($_SESSION['username']) && isset($_SESSION['password'])) { header("Location: private.php"); } ?> <html> <head> <title>Login Page</title> </head> <body> <?php if (isset($_POST['uname']) && isset($_POST['pass'])) { if (!empty($_POST['uname']) && !empty($_POST['pass'])) { $uname=stripslashes(trim($_POST['uname'])); $pass=stripslashes(trim($_POST['pass'])); mysql_connect("MySQL Host","Username","password"); mysql_select_db("users"); $check=mysql_query("SELECT * FROM login WHERE username='$uname' AND password='$pass'"); if(mysql_num_rows($check)!=0) { $details=mysql_fetch_array($check); $_SESSION['display_name']=$details[0]; $_SESSION['username']=$details[1]; $_SESSION['password']=$details[2]; header("Location: private.php"); } else { print "Invalid Username/Password"; } } else { print "All fields must be filled"; } } else { ?> <form action="login.php" method="post"> Username: <input type="text" name="uname" /><br /> Password: <input type="password" name="pass" /><br /> <input type="submit" value="Login" /> </form> <?php } ?> </body> </html>
In the above source code notice how HTML tags are used above and below the PHP code if you want to use them inside the PHP tags put them inside a print or echo statement. While doing so if there are double quotes in the HTML syntax you should use the escape character \” to specify them else they will conflict with the PHP syntax. Example to print <p align=”center”>Paragraph</p> it should be written as print “<p align=\”center\”>Paragraph</p>”; To avoid all the confusion you can close the PHP tag ?> just before the HTML code begins and reopen it again to continue the PHP script.
User Control Panel
All through this article a file named private.php has been specified inside the code. This will be a simple user control panel through which the user can change his/her password, display name, delete the user account and logout. We’ll see this one by one. The first objective is to welcome the user by greeting them by their name. So the starting of private.php is
session_start(); if(isset($_SESSION['username']) && isset($_SESSION['password'])) { ?> <h3>Welcome <?php print $_SESSION['display_name']; ?></h3> <?php } else { header("Location: login.php"); }
The session_start() function doesn’t start a new session here it resumes the already logged in session. The if loop checks whether the user is logged in and proceeds to display the name of the user else it redirects to the login page. Now lets create links to do the task of managing the user account. Four new links are added in an unordered list.
<?php session_start(); if(isset($_SESSION['username']) && isset($_SESSION['password'])) { ?> <h3>Welcome <?php print $_SESSION['display_name']; ?></h3> <ul> <li><a href="change_name.php">Change Display Name</a></li> <li><a href="change_pass.php">Change Password</a></li> <li><a href="logout.php">Log out</a></li> <li><a href="delete_account.php">Delete Account</a></li> </ul> <?php } else { header("Location: login.php"); } ?>
Now lets create the files specified in the anchor tag one by one. The first one is to change the display name. For this we need to use the update query in the mysql_query() function and create a HTML form, place it inside the PHP code in an if else loop as we did earlier. Before that make sure you have an if loop which redirects the user to the login page if he is not logged in
<?php session_start(); if(!isset($_SESSION['username']) || !isset($_SESSION['password'])) { header("Location: login.php"); } if(isset($_POST['new_name'])) { if(!empty($_POST['new_name'])) { $old_name=$_SESSION['display_name']; $new_name=stripslashes(trim($_POST['new_name'])); $uname=$_SESSION['username']; mysql_connect("MySQL_Host","Username","Password"); mysql_select_db("users"); mysql_query("UPDATE login SET full_name='$new_name' WHERE username='$uname'") or die(mysql_error()); $_SESSION['display_name']=$new_name; print "Your Account name has been changed from $old_name to $new_name"; } else { print "Field cannot be blank"; } } else { ?> <form action="change_name.php" method="post"> Current Display Name is <b><?php print $_SESSION['display_name']; ?></b><br /> Enter a new display name: <input type="text" name="new_name" /><br /> <input type="submit" value="change" /> </form> <?php } ?> <a href="private.php">Go Back</a>
The next module is the “Change Password” module. Here we’ll have three fields current password, new password, retype new password and is both the new passwords match the password will be changed using the UPDATE query.
<?php session_start(); if(!isset($_SESSION['username']) || !isset($_SESSION['password'])) { header("Location: login.php"); } if (isset($_POST['cur_pass']) && isset($_POST['pass1']) && isset($_POST['pass2'])) { if (!empty($_POST['cur_pass']) && !empty($_POST['pass1']) && !empty($_POST['pass2'])) { $current_pass=stripslashes(trim($_POST['cur_pass'])); $pass1=stripslashes(trim($_POST['pass1'])); $pass2=stripslashes(trim($_POST['pass2'])); if($pass1==$pass2) { if($current_pass==$_SESSION['password']) { mysql_connect("localhost","root","JESIN"); mysql_select_db("users"); mysql_query("UPDATE login SET password='$pass1' WHERE username='".$_SESSION['username']."'"); $_SESSION['password']=$pass1; print "Password successfully changed"; } else { print "The current password is incorrect"; } } else { print "Both the passwords do not match"; } } else { print "Please fill all the fields"; } } else { ?> <form action="change_pass.php" method="post"> Current Password: <input type="password" name="cur_pass" /><br /> New Password: <input type="password" name="pass1" /><br /> Retype New Password: <input type="password" name="pass2" /><br /> <input type="submit" value="Change Password" /> </form> <?php } ?>
Even though the code may look complicated it mainly consists of conditional statements which check whether the correct password is entered and both the passwords match. The next task is to create a logout page. This is the simplest one and uses just a session_destroy() function to destroy all the session variables and then redirects the user to the login form.
<?php session_start(); session_destroy(); header("Location: login.php"); ?>
The final module is the delete account module which will remove the user’s record from the database using the delete query. Before deleting the account the user has to confirm the deletion.
<?php session_start(); if(!isset($_SESSION['username']) || !isset($_SESSION['password'])) { header("Location: login.php"); } if(isset($_POST['confirm'])) { if($_POST['confirm']=='Yes') { mysql_connect("localhost","root","JESIN"); mysql_select_db("users"); mysql_query("delete from login where username='".$_SESSION['username']."'"); print "Your account has been deleted"; session_destroy(); } else { header("Location: private.php"); } } else { ?> <form method="post" action="del_account.php"> <h4>Are you sure you want to delete your account ?</h4> <input type="submit" value="Yes" name="confirm" /> <input type="submit" value="No" name="confirm" /> </form> <?php } ?>
Download PHP Login Script
The entire script is available for download. Download it edit the db_info.php file and enter your database details. Then execute the create_db_table.php to create the necessary database with a table. Now you can implement the modules to your website.
I love your wp format, where did you get a hold of it?
Are you referring to the theme? It is a Genesis child theme developed by me. The code snippets are formatted by the SyntaxHighlighter plugin.
Hello, I wanted to inform people who are reading this article that MySQL extension is nolonger available for the latest version of PHP so yo need to choose between MySQLi and PDO, here is an article that explains how to use PDO when you want to insert, update or delete
http://www.mustbebuilt.co.uk/php/insert-update-and-delete-with-pdo/
You also need to use bindParam which is a method of a PDOStatement object after calling prepare() method of the PDO object, bindParam is very usefull to protect against sql injection