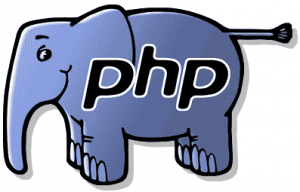
This PHP sessions tutorial explains how sessions work, commonly used PHP session handling functions and how to use then. A session is the time duration that starts from the time a user visits a website and ends when he/she leaves the website. Usually when we use PHP variables they can be accessed only in the script in which they are used but sessions variables can be accessed by all PHP scripts which are accessed during that “session” Sessions greatly help in personalizing an user’s experience on a website, they can also be used to create Login pages using PHP. We’ll first see how PHP sessions work.
How do PHP sessions work ?
When a session is started by a PHP script a session cookie is sent to the client a.k.a. browser requesting the script. This session cookie named PHPSESSID by default contains a 32 character “session” ID which is set to expire when the session ends.
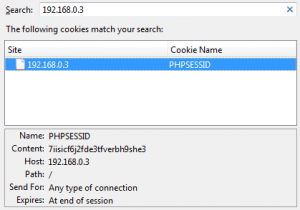
On the server-side a file named sess_X (replace X with the 32 character session ID) is created in the location specified in session.save_path setting in the php.ini file. This is C:\WINDOWS\Temp for windows and /var/lib/php/session in Linux. This file contains the names of all session variables and their respective values. So when a user whose browser contains the session ID 7iisicf6j2fde3tfverbh9she3 accesses the website the server checks for the file named sess_7iisicf6j2fde3tfverbh9she3 in its session save path and accordingly uses the variables in that file.
PHP Session Functions
Some of the commonly used session functions are explained here
session_start() This function starts a session or resumes a session if it already exists. This should be used at the beginning of any script which uses a session. If this function is not called none of the session variables can be accessed. When this function is called for the first time a cookie is set named PHPSESSID (default name) containing the session ID.
session_id() This function sets or the current session ID. If this function is called without any parameters it returns the current session ID. If a string is specified session_id(“jb4jkjk4b3j6basd”) it will set the session ID to the one specified.
session_name() This function sets or gets the session name. The default name is PHPSESSID if this function is called without parameters it returns the current session name. If a string is passed as a parameter it is set as the session name session_name(“WebsiteSess”) To set a session name this function should be called before the session_start() function.
session_destroy() This destroys all session variables. This function is mainly used in logout pages to delete all session variables. The session_destroy() must be called AFTER the session_start() function else variables will NOT be removed.
$_SESSION This is not a function it is a global array variable which contains all session variables and their values. Session variables are created by assigning values to the index of this variable. e.g $_SESSION[‘username’]=”Jesin” The index value of $_SESSION is user defined.
An example for PHP Sessions
Lets create a small script spanning over 3 pages which outline how sessions can be used to pass data between pages.
<?php session_start(); print "Session start. Session variable is " . session_id() . " and session name is " . session_name(); $_SESSION['name']="Jesin"; print "The session variable 'name' contains " . $_SESSION['name']; ?>
<?php session_start(); if(isset($_SESSION['name'])) { print "Your name is " . $_SESSION['name']; } else { print "You directly visited this page"; } ?>
<?php session_start(); print "The session variable name contains ".$_SESSION['name']; print "<br>We'll now destroy all variables"; session_destroy(); print "<br>The session variable name contains ".$_SESSION['name']; ?>
Create these three pages when you visit the first page a session is created, its ID and name are displayed a session variable named “name” is created with value “Jesin” When you visit Page2.php it checks whether the session variable “name” is set and displays the name assigned in the first page. Now visiting the third page will display the value of the variable first and then destroy it. Now when it displays the session variable again nothing is shown. Now go to Page2.php you’ll see the line “You directly visited this page” because the session variables are destroyed.
Some things to take note while using sessions. All scripts using sessions must start with the function session_start() including the script which destroys the session. Any number of session variables can be defined using $_SESSION variable. For more PHP session functions see http://php.net/manual/en/ref.session.php
Leave a Reply