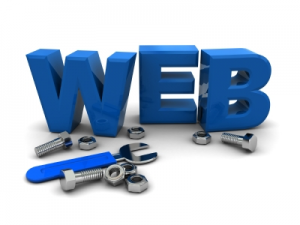
Recently the AJAX bug bit me and I started rewriting the code of htdigest generator, htpasswd generator and URL encoder/decoder after learning some basics from w3schools.com. I tried creating a username availability validator using AJAX and it worked well so I am here writing this article on how to do this. I will be concentrating more on the AJAX part and less on PHP because I have already written an article on how to connect PHP and MySQL. In the end of this article there is a link to a demo which I created, it has a HTML form sans form tags 😛 just to demonstrate username validation.
AJAX Code
First design a basic HTML form with a <span> tag next to the username input box to display validation messages. The <span> tag should have an ID attribute so that it can be referenced in javascript.
<div>Username: <input type="text" maxlength="10" name="uname" id="uname" /><span id="status"></span></div>
I’m covering only the line which validates the username and not the form handling and other stuff. Paste the following code somewhere under the form.
<script type="text/javascript"> document.getElementById("uname").onblur = function() { var xmlhttp; var uname=document.getElementById("uname"); if (uname.value != "") { if (window.XMLHttpRequest) { xmlhttp=new XMLHttpRequest(); } else { xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("status").innerHTML=xmlhttp.responseText; } }; xmlhttp.open("GET","/uname_availability.php?uname="+encodeURIComponent(uname.value),true); xmlhttp.send(); } }; </script>
Lets dive deep into the code and understand its working.
document.getElementById("uname").onblur = function()
The first line triggers the entire function when the element with ID “uname” looses focus i.e. when the cursor goes inside the text box and then leaves it. The next two lines are variable declarations which need no explanation. Moving to the first “if” statement.
if (uname.value != "")
This line ensures validation is not unnecessarily performed if the text box is left empty. Now lets get into the heart of the code.
if (window.XMLHttpRequest) { xmlhttp=new XMLHttpRequest(); } else { xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); }
These lines are used to create a HTTP request object. If statement is true on browsers IE7+, Firefox, Chrome, Opera, Safari the else loop is executed if the browser is IE5 or 6 and an ActiveX object is created.
xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("status").innerHTML=xmlhttp.responseText; } }; xmlhttp.open("GET","/uname_availability.php?uname="+encodeURIComponent(uname.value),true); xmlhttp.send();
The xmlhttp.onreadystatechange is an event which is triggered after xmlhttp.send sends a request. The xmlhttp.open statement sends a GET request to the PHP URL mentioned and passes the username entered as a query string. If the server returns a status code of 200 it is matched by the “if” statement xmlhttp.status and the response text is placed in the innerHTML of the element with ID “status” (which is <span> tag in this case).
PHP Code
Now the PHP code has to GET this data match it with the database and print an answer. The following code will do just that.
<?php $uname=$_REQUEST['uname']; if(preg_match("/[^a-z0-9]/",$uname)) { print "<span style=\"color:red;\">Username contains illegal charaters.</span>"; exit; } mysql_connect("localhost","Username","Password"); mysql_select_db("database_name"); $data=mysql_query("SELECT * FROM users where username='$uname'"); if(mysql_num_rows($data)>0) { print "<span style=\"color:red;\">Username already exists :(</span>"; } else { print "<span style=\"color:green;\">Username is available :)</span>"; } ?>
See how it works on this demo page. Do post your comments and suggestions. Check these articles for more javascript validations.
This is open to MySQL SQL Injection.
Should be
i have tried your code for checking username availability but there is one issue according to my website as per your example it works fine but when i select existing user name from database it shows message like user name is exist but even if this message is shown when i click on submit button it stores data in database so i want to know how make validation that not allow user to store existing user name when he/she click on submit button.
thanks
Use something like:
document.getElementById(‘submit’).disabled = true;
This will disable the submit button. After checking whether it is available, you could enable it by changing ‘true’ to ‘false’.
Thanq the code is very helpful.
thank you …
You could use jquery for the ajax call its much simple. Anyway code is working perfect. Thanks Alot