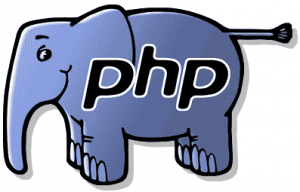
Create a PHP captcha using simple PHP image handling functions. Creating a PHP Captcha image explained. The only difference between a captcha and a normal text written on an image is the former generates random strings and places it crookedly on the image making it difficult for bots to find out. This PHP captcha tutorial will make use of six image functions imagecreate(), imagecolorallocate(), imageline(), imagettftext(), imagepng(), imagedestroy(). You can feel free to modify it and add more functions to bring visual effects. For PHP Random string generation see the previous article.
First thing is to create a image of dimensions 200×100 using the imagecreate() function. You can choose any size. This image should be allotted some color this is done using the imagecolorallocate() function in RGB format. This image must be formatted to PNG format so imagepng() function is used. When this script is accessed through the browser the headers send should tell it is a PNG image the header() function does this. After this script is run the memory should be freed up of this image so imagedestroy() does this.
<?php $captcha=imagecreate(200,100); imagecolorallocate($captcha,0,0,0); header ("Content-type: image/png"); imagepng($captcha); imagedestroy($captcha); ?>
The code above will create a 200×100 image with black background. Now using the imagettftext() function a randomly generated string is written to the image. When using this function a true type font file location has to be specified. For more flexibility copy the font file to the location of your PHP script. Or you can specify the absolute paths. For windows OSes use C:/WINDOWS/Fonts/font_name.ttf (note the use of forwardslash in the location path) and for Linux /usr/share/fonts/true-type-font. The syntax of imagettftext() is
imagettftext(image,size,andle,x-axis,y-axis,color identifier,fontfile);
The following code illustrates the usage in windows
<?php $captcha=imagecreate(200,100); imagecolorallocate($captcha,0,0,0); imagettftext($captcha,30,0,30,60,imagecolorallocate($captcha,255,255,255),"C:/WINDOWS/Fonts/ARIAL.TTF",randomstring(6)); header ("Content-type: image/png"); imagepng($captcha); imagedestroy($captcha); ?>
Now we come to the important part, randomizing the x-axis, y-axis, angle and color values for each character. So the random string will be assigned to a variable and each character will be written to the image with random attributes. You can choose what attributes to randomize
<?php $text=randomstring(6); $captcha=imagecreate(200,100); imagecolorallocate($captcha,0,0,0); for($i=0;$i<strlen($text);$i++) { $randcolors = imagecolorallocate($captcha,rand(100,255),rand(200,255),rand(200,255)); imagettftext($captcha,30,rand(-30,30),10+30*$i,rand(40,70),$randcolors,"C:/WINDOWS/Fonts/ARIAL.TTF",$text[$i]); } header ("Content-type: image/png"); imagepng($captcha); imagedestroy($captcha); ?>
Here the size of the font is fixed at 30 but if you’re a bit adventurous you can also randomize it. You can use the captcha at this stage but to make it difficult for the bad guys (read machines) we’ll draw a few random line all over the image. A for loop will be used to generate twenty gray colored lines on the image. Their x and y axises will be random values limited between 0 and 100
<?php $text=randomstring(6); $captcha=imagecreate(200,100); imagecolorallocate($captcha,0,0,0); $gray=imagecolorallocate($captcha,128,128,128); for($i=0;$i<10;$i++) { imageline($captcha,rand(0,10)*20,0,rand(0,10)*20,100,$gray); imageline($captcha,0,rand(0,10)*10,200,rand(0,10)*10,$gray); } for($i=0;$i<strlen($text);$i++) { $randcolors = imagecolorallocate($captcha,rand(100,255),rand(200,255),rand(200,255)); imagettftext($captcha,30,rand(-30,30),10+30*$i,rand(40,70),$randcolors,"C:/WINDOWS/Fonts/ARIAL.TTF",$text[$i]); } header ("Content-type: image/png"); imagepng($captcha); imagedestroy($captcha); ?>
the captcha has been successfully generated but how to verify whether the user entered value matches with the text ? The answer is to store the generated text in a session variable in an encrypted format and then verify it. So now the captcha code is
<?php session_start(); $text=randomstring(6); $_SESSION['captcha_text']=md5(strtolower($text)); $captcha=imagecreate(200,100); imagecolorallocate($captcha,0,0,0); $gray=imagecolorallocate($captcha,128,128,128); for($i=0;$i<10;$i++) { imageline($captcha,rand(0,10)*20,0,rand(0,10)*20,100,$gray); imageline($captcha,0,rand(0,10)*10,200,rand(0,10)*10,$gray); } for($i=0;$i<strlen($text);$i++) { $randcolors = imagecolorallocate($captcha,rand(100,255),rand(200,255),rand(200,255)); imagettftext($captcha,30,rand(-30,30),10+30*$i,rand(40,70),$randcolors,"C:/WINDOWS/Fonts/ARIAL.TTF",$text[$i]); } header ("Content-type: image/png"); imagepng($captcha); imagedestroy($captcha); ?>
Notice that the text stored in the session variable is converted to lowercase using strtolower() function. The code for the page showing the captcha with a text box.
<html> <head> <title>Captcha Verification</title> </head> <body> <form action="" method="post"> <img src="captcha.php" /> <br /><input type="text" name="captcha_txt" /> <input type="submit" value="verify" /><br /> <?php session_start(); if(isset($_POST['captcha_txt'])) { if($_SESSION['captcha_text']==md5(strtolower($_POST['captcha_txt']))) { print "<span style=\"color:green\">Correct! You are human</span>"; } else { print "<span style=\"color:red\">Incorrect Are you a machine ?</span>"; } } ?> </form> </body> </html>
To refresh this CAPTCHA image read Javascript Reload Image. Download PHP captcha script. Try out a demo
Leave a Reply