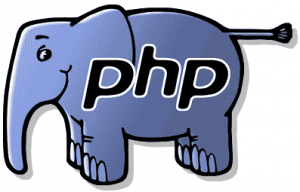
Generate a random alphanumeric string containing both uppercase and lowercase characters in PHP. The rand() function in PHP generates a random number we’ll be using this function to generate a random alphanumeric string. A variable should be declared containing all the alphabets in English both uppercase and lowercase (A-Z and a-z) and numbers from 0-9. The concept here is to randomly pick a character from the string containing all the characters and assign it to a variable. Each time this process is done the currently randomly selected character is concatenated with the previously generated characters. This should be done as many times the length of the random string required. For this a looping statement can be used.First lets assign all the uppercase and lowercase alphabets and numbers to a variable named $chars. Then the variable which will hold the random string value should be assigned an empty value.
$chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; $string = "";
If you don’t assign $string and empty value you’ll get a notice saying “Notice: Undefined variable: string in /path/to/php/file on line xx” if you have display_errors on. Using the substr() function a single character is randomly picked from $chars variable. The syntax for substr() is substr(string_variable,start_position,length). In this case the string variable is $chars and length is 1 the start_position parameter should be randomly generated using rand() function. So the min value parameter of rand() is 0 and max value is the length of all the characters. The value returned should be assigned to $string and st the same time concatenated with the previous values. So it will look like $string = $string . substr(parameters) instead we can shortly write it as $string .= substr(parameters) All this should be placed inside a for loop which should loop the length of random string required.
$string = ""; $chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; for($i=0;$i<10;$i++) $string.=substr($chars,rand(0,strlen($chars)),1);
The above code will generate a random string containing 10 characters and store it inside $string. To create a PHP function generating random string the following code is used.
function randomstring($len) { $string = ""; $chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; for($i=0;$i<$len;$i++) $string.=substr($chars,rand(0,strlen($chars)),1); return $string; }
The function should be called with a integer as a length parameter. E.g. randomstring(20) will return a random string containing 20 characters.
Leave a Reply