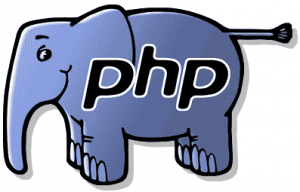
This tutorial explains how to use checkboxes in PHP to get values which are checked by the user. Use checkboxes in PHP and insert the values into MySQL. Usually using other HTML form controls with PHP is easy. The input element is assigned a name and values entered can be retrieved using GET or POST method. But in the case of checkboxes it might be ticked or left empty also. Using checkboxes in PHP involves setting the name attribute of all checkboxes to a common name with an empty square bracket denoting an array. When the form is submitted the vales of the items checked are send as an array which is explained here
First create checkboxes inside the HTML form and name them accordingly. A sample is shown below
<input type="checkbox" name="cbox[]" value="Item 1" />Item 1 <input type="checkbox" name="cbox[]" value="Item 2" />Item 2 <input type="checkbox" name="cbox[]" value="Item 3" />Item 3 <input type="checkbox" name="cbox[]" value="Item 4" />Item 4 <input type="checkbox" name="cbox[]" value="Item 5" />Item 5
Now getting the checkbox value in PHP is very easy. The values of the checked checkboxes are stored in the array cbox. So in the PHP you’ll retrieve them as $_POST[‘cbox’][index] or $_GET[‘cbox’][index] or $_REQUEST[‘cbox’][index] we’ll use the implode() function to glue together all checked values to display them
<?php $items = implode(",",$_REQUEST['cbox']); print "You selected $items"; ?>
The output of the above code will be “You selected item 2,Item 4,Item 5” provided the user checked the Items 2, 4 and 5. You can also insert the values into the MySQL database using PHP in the same way as a complete string with values separated by commas. For example you want to enter the hobbies of a student into the MySQL database.
<?php $hobbies = implode(",",$_REQUEST['hobbies']); mysql_query("INSERT INTO `table_name` (hobbies) VALUES ('$hobbies')"); ?>
To retrieve them from the database and display as a list use the explode() function to separate the values and display them through foreach statement.
<?php $hobbies = mysql_fetch_array(mysql_query("SELECT `hobbies` FROM `table_name` WHERE name='jesin'")); $hobby = explode(",",$hobbies); print "<ul>"; foreach ($hobby as $item) { print "<li>$item</li>"; } print "</ul>"; ?>
See a live demo of using checkboxes in PHP. Select the films of your choice and you’ll see them as a list.
Thanks for share Jesin, good job.
can you give an example how to email item 2,3,5….. ONLY
Easy lesson bro. Thanks for saving my day!
Thank you so much for your help.