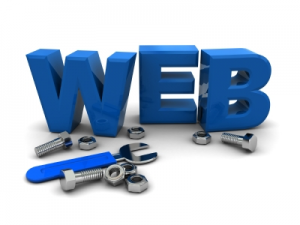
Validate your HTML form with Javascript validation. The tutorial describes Javascript validation of textboxes, comboboxes, radio buttons and checkboxes. In Javascript we create a function which checks if some value is entered in the textbox or selected in the case of combobox, radiobutton, checkbox. If a textbox is empty or an option is not selected we can use javascript to display an alert message, and highlight the empty field and also we should make sure the page stays the same and doesn’t get “submitted”
Disclaimer: Javascript validation is a client side validation and relies on the web browser so it should not be solely relied upon
Javascript Textbox Validation
Textbox is the simplest element to validate if empty or not. We need to check if it is equal to null or “” because different browsers act differently so its better to check for both the conditions. The following function checks whether the texbox with id name1 is empty, if empty it displays a message and highlights that textbox with red color.
<script type="text/javascript"> function validate() { var txt = document.getElementById("name1"); if(txt.value== "" || txt.value== null) { alert("Please enter a name"); txt.style.border = "2px solid red"; return false; } else { txt.style.border = ""; } } </script> <form action="" method="post" onsubmit="return validate()"> Name: <input type="text" name="name1" id="name1" /> <input type="submit" value="Submit" /> </form>
We use return false; because when this function is called when the form is submitted and if this textbox is found empty the page shouldn’t be submitted so returning the boolean value false with prevent the form from being submitted.
Javascript Combobox Validation
An HTML combobox shows a dropdown list of items from which one item is selected. By default the caption for that list is the first item. For example if you’re asking a user to select a country the first option is “Select a country” and the name of the countries follow so we need to check if the first option is selected and then display a message to select an option.
<script type="text/javascript"> function validate() { var combo1 = document.getElementById("country") if(combo1.value == null || combo1.value == "") { alert("Please select a country"); document.getElementById("cty").style.border = "2px solid red"; return false; } else { document.getElementById("cty").style.border = ""; } } </script> <form action="" method="post" onsubmit="return validate()"> <span id="cty"> Country: <select name="country" id="country"> <option value="">Choose a country</option> <option value="aus">Australia</option> <option value="gb">Great Britain</option> <option value="ind">India</option> </select> </span> <input type="submit" value="Submit" /> </form>
Notice that the value attribute for the first option is empty if you set it to something else do the same in the if condition in Javascript.
Javascript Radiobutton validation
A radio button allows the user to select only one option so Javascript should display a message only if none of the radiobuttons are selected. This is a bit tricky first we need to check if none of the radiobuttons are checked then display a message. For this we use a variable which is incremented each time a radiobutton is NOT checked then it is compared with the number of radiobuttons in that group, if both are equal a message is displayed.
<script type="text/javascript"> function validate() { var a = 0, rdbtn=document.getElementsByName("gender") for(i=0;i<rdbtn.length;i++) { if(rdbtn.item(i).checked == false) { a++; } } if(a == rdbtn.length) { alert("Please select your gender"); document.getElementById("gen").style.border = "2px solid red"; return false; } else { document.getElementById("gen").style.border = ""; } } </script> <form action="" method="post" onsubmit="return validate()"> <span id="gen"> Gender: <input type="radio" name="gender" value="male"> Male <input type="radio" name="gender" value="female"> Female </span> <input type="submit" value="Submit" /> </form>
Javascript Checkbox Validation
In the case of a checkbox multiple values can be selected so validation is done if none of the checkboxes are ticked. You can also validate by requiring the user to select a minimum of one value or two value according to your requirement. The first example shows how to validate a checkbox if nothing is checked. This is similar to radiobutton example explained above.
<script type="text/javascript"> function validate() { var b = 0, chk=document.getElementsByName("cars") for(j=0;j<chk.length;j++) { if(chk.item(j).checked == false) { b++; } } if(b == chk.length) { alert("Please select a car"); document.getElementById("crs").style.border = "2px solid red"; return false; } else { document.getElementById("crs").style.border = ""; } } </script> <form action="" method="post" onsubmit="return validate()"> <span id="crs"> Select the cars you own: <input type="checkbox" name="cars" value="Toyota">Toyota <input type="checkbox" name="cars" value="Ferrari">Ferrari <input type="checkbox" name="cars" value="Lamborghini">Lamborghini </span> <input type="submit" value="submit" /> </form>
Now for the second example in this situation you want the user to check a minimum number of checkboxes. This example will force the user to select a minimum of two checkboxes
<script type="text/javascript"> function validate() { var b = 0, chk=document.getElementsByName("cars") for(j=0;j<chk.length;j++) { if(chk.item(j).checked == false) { b++; } } if(b+1 >= chk.length) { alert("Minimum of two cars should be selected"); document.getElementById("crs").style.border = "2px solid red"; return false; } else { document.getElementById("chkgrp").style.border = ""; } } </script> <form action="" method="post" onsubmit="return validate()"> <span id="crs"> Select the cars you own: <input type="checkbox" name="cars" value="Toyota">Toyota <input type="checkbox" name="cars" value="Ferrari">Ferrari <input type="checkbox" name="cars" value="Lamborghini">Lamborghini </span> <input type="submit" value="submit" /> </form>
Take note of the condition which is bold, the value 1 which is added to the variable b is the number of checkboxes to be checked minus one, so to have a minimum of one value checked user b >= chk.length and for three checkboxes b+2 >= chk.length
See a demo of Javascript Validation
Question.
What is I have two sets of radio buttons, one after the other?
For example:
Gender: [] Male [] Female
League Member: [] Yes [] No
How would the code look then? Can’t seem to figure it out myself.
Thanks!
Check the code under “Javascript Radiobutton validation”. Use another set of variables to get the value of the second set of options like
document.getElementsByName("leaguemember")
and check if its length is 0.