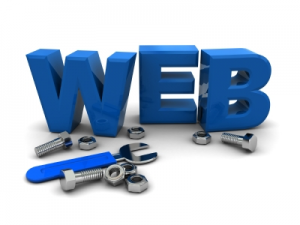
After reading this article you’ll be able to do advanced javascript validation using regular expressions a.k.a. RegExp for basic javascript validation read Javascript Validation – Textbox Combobox Radiobutton Checkbox. Using regular expressions the entered content can be validated with advanced conditions like the string entered shouldn’t contain spaces, special characters, numbers, uppercase or lowercase etc. Once you learn the art of using regular expressions you can use it with many computer languages.
Regular Expressions
The following is how regular expressions can be used
[abc] – find any character in the brackets a, b or c
[a-z] – find the range of characters within brackets i.e. a to z lowercase. Can also be used with numbers [0-9]
[^xyz] – find any character other than the ones specified in the brackets i.e. x,y and z
(word) – find the “word” specified in the round brackets
[abc|xyz] – find either the characters a,b,c or x,y,z
Javascript Validation Numeric
We’ll start first with validating a textbox that accepts only numbers. So the condition is we check whether the textbox contains anything ELSE other than numbers and display a message
<script type="text/javascript"> function validate() { var regexp1=new RegExp("[^0-9]"); if(regexp1.test(document.getElementById("age").value)) { alert("Only numbers are allowed"); return false; } } </script> <form action="" method="post" onsubmit="return validate()"> <input type="text" id="age" name="age"> <input type="submit" value="submit"> </form>
As you can see the function validate() checks if the entered string contains characters that does NOT (notice the ^ symbol) match the numbers 0 to 9 including white spaces and special characters.
Javascript Validation Alphabets
From numbers we’ll move to alphabets first an example for validation lowercase alphabets and then to validate both uppercase and lowercase.
<script type="text/javascript"> function validate() { var regexp1=new RegExp("[^a-z]"); if(regexp1.test(document.getElementById("txt").value)) { alert("Only alphabets from a-z are allowed"); return false; } } </script> <form action="" method="post" onsubmit="return validate()"> <input type="text" id="txt" name="txt"> <input type="submit" value="submit"> </form>
Now we’ll use the pipe symbol (|) to check if the value is outside a-z OR A-Z.
<script type="text/javascript"> function validate() { var regexp1=new RegExp("[^a-z|^A-Z]"); if(regexp1.test(document.getElementById("txt").value)) { alert("Only alphabets from a-z and A-Z are allowed"); return false; } } </script> <form action="" method="post" onsubmit="return validate()"> <input type="text" id="txt" name="txt"> <input type="submit" value="submit"> </form>
The above code will reject even whitespaces but in case you want to allow whitespaces the following code will do it.
<script type="text/javascript"> function validate() { var pattern=/[^a-z|^A-Z|^\s]/; if(document.getElementById("txt").value.match(pattern)) { alert("Only alphabets from a-z and A-Z are allowed"); return false; } } </script> <form action="" method="post" onsubmit="return validate()"> <input type="text" id="txt" name="txt"> <input type="submit" value="submit"> </form>
Here we create a RegExp pattern and use the match to validate it.
Javascript validation check for a word
This type of javascript validation will check if the entered text contains a particular word round brackets are used to find out complete words
<script type="text/javascript"> function validate() { var regexp1=new RegExp("(word1|word2)"); if(regexp1.test(document.getElementById("txt").value)) { alert("Word found in string"); return false; } } </script> <form action="" method="post" onsubmit="return validate()"> <input type="text" id="txt" name="txt"> <input type="submit" value="submit"> </form>
Two words are checked in this code word1 and word2 for checking a single word use new RegExp(“(word)”)
Javascript Validation whitespace
Validate a string against any whitespaces including tab and carriage return using the pattern and match function
<script type="text/javascript"> function validate() { var pattern=/\s/; if(document.getElementById("txt").value.match(pattern)) { alert("Whitespaces are not allowed"); return false; } } </script> <form action="" method="post" onsubmit="return validate()"> <input type="text" id="txt" name="txt"> <input type="submit" value="submit"> </form>
Very nice article given and easily help for anyone !!!
very good.My knowledge has improved.
need a code for limiting the entry of special symbol in description field.
nice one……….
very good.My knowledge has improved.
Nice One.. Thank you…