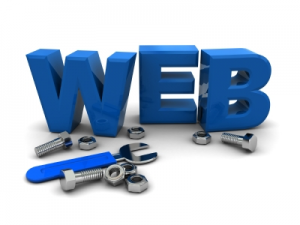
Create a Simple slideshow using Javascript and HTML which is very easy to understand and implement. This is a no frills slideshow without any special effects and aims at beginners. As you learn more you can add more special effects. The Javascript Simple Slideshow works by changing the src attribute of the img tag to the list of image locations specified in an array variable. And finally I’ll show you how to preload the images so that the transition takes place smoothly.
Lets go step-by-step building this Simple Slideshow using Javascript. I assume you are familiar with the basics of HTML and Javascript
Step 1: Create the HTML Code
Type the HTML code with an img tag showing an image. The img tag should have an id attribute
<html> <head> <title>Simple Javascript Slideshow</title> </head> <body> <img id="slideshow" src="photos/photo1.jpg" /> </body> </html>
The id attribute you specify here will be used by Javascript to modify other attributes of this img element.
Step 2: Add a Javascript Array with images list
Inside the head tag create a script tag with an array variable containing list of image locations.
<html> <head> <title>Simple Javascript Slideshow</title> <script type="text/javascript"> var imgsrc = new Array(); imgsrc[0]="photos/photo1.jpg"; imgsrc[1]="photos/photo2.jpg"; imgsrc[2]="photos/photo3.jpg"; imgsrc[3]="photos/photo4.jpg"; imgsrc[4]="photos/photo5.jpg"; imgsrc[5]="photos/photo6.jpg"; </script> </head> <body> <img id="slideshow" src="photos/photo1.jpg" /> </body> </html>
The locations specified in the array should be relative to the location where this file is placed. You can add as many images you want by expanding the array. But make sure all the images are of the same size else specify the width and height attribute for the img tag <img id=”slideshow” src=”photos/photo1.jpg” width=”400″ height=”300″ /> If this isn’t specified and you used images of various sizes the page will expand and contract during the slideshow making it look ugly.
Step 3: Create a function to start the Slideshow
We’ll now create a function inside which the src attribute of the img element with id slideshow will be modified. Declare a counter variable which will change the array index of the variable imgsrc at each interval. So we should first initialize the value of of that variable to 0 then it should be incremented each time this function is called. But we’ll face a problem after the counter variable is incremented to value greater than the size of the array. This can be rectified by using an if loop to check whether the value of the counter variable is equal to the size of the array. If so it will be assigned 0 so that it can start again. An inbuilt function named setTimeout will keep calling the slideshow function at regular time intervals.
<html> <head> <title>Simple Javascript Slideshow</title> <script type="text/javascript"> var i = 0, imgsrc = new Array(); imgsrc[0]="photos/photo1.jpg"; imgsrc[1]="photos/photo2.jpg"; imgsrc[2]="photos/photo3.jpg"; imgsrc[3]="photos/photo4.jpg"; imgsrc[4]="photos/photo5.jpg"; imgsrc[5]="photos/photo6.jpg"; function startSlideshow() { document.getElementById("slideshow").src=imgsrc[i]; i++; if(i==imgsrc.length) { i=0; } setTimeout("startSlideshow()",1000); } </script> </head> <body> <img id="slideshow" src="photos/photo1.jpg" /> </body> </html>
The value 1000 given as a parameter of the function setTimeout indicates the interval time in milliseconds so every one second the function startSlideshow() will be called. The value of the interval can be increased as per requirement.
Step 4: Preload the images
If you start the slideshow at this point the first time it executes it’ll take sometime to load each image as it is called so the transition will not be seamless. To rectify this we’ll preload all the images before the slideshow starts.
<html> <head> <title>Simple Javascript Slideshow</title> <script type="text/javascript"> var i = 0, imgsrc = new Array(), preload = new Array(); imgsrc[0]="photos/photo1.jpg"; imgsrc[1]="photos/photo2.jpg"; imgsrc[2]="photos/photo3.jpg"; imgsrc[3]="photos/photo4.jpg"; imgsrc[4]="photos/photo5.jpg"; imgsrc[5]="photos/photo6.jpg"; for (var j=0;j<imgsrc.length;j++) { preload[j] = new Image; preload[j].src = imgsrc[j]; } function startSlideshow() { document.getElementById("slideshow").src=imgsrc[i]; i++; if(i==imgsrc.length) { i=0; } setTimeout("startSlideshow()",1000); } </script> </head> <body> <img id="slideshow" src="photos/photo1.jpg" /> </body> </html>
A new array variable named preload is declared and each image is called inside the for loop thus loading it in the visitor’s system. You can stop at this point and call the startSlideshow() function inside the body onload event <body onload=”startSlideshow()”> To add more functionality read the last step.
Step 5: Play, Pause and Stop the slideshow
The final touch is to add three buttons which will play, pause and stop the slideshow. This will involve creating a function which will accept the mode as a parameter and assign it to a variable. Inside the startSlideshow() function the value of this variable will be checked using else if loops and accordingly the action will happen. To make things more professional the pause and stop buttons will be disabled when the page loads, after pressing the play button the other two buttons will be enabled and the play button will be greyed out. Clicking the Pause button will enable and change the value of Play button to Resume. Clicking Stop will set the value of the Resume button to play, enable it and disable itself and the Pause button. The final code is here
<html> <head> <title>Simple Javascript Slideshow</title> <script type="text/javascript"> var i = 0, imgsrc = new Array(), preload = new Array(); imgsrc[0]="photos/photo1.jpg"; imgsrc[1]="photos/photo2.jpg"; imgsrc[2]="photos/photo3.jpg"; imgsrc[3]="photos/photo4.jpg"; imgsrc[4]="photos/photo5.jpg"; imgsrc[5]="photos/photo6.jpg"; for (var j=0;j<imgsrc.length;j++) { preload[j] = new Image; preload[j].src = imgsrc[j]; } function mode(param) { smode=param; } function startSlideshow() { if(smode=="play") { document.getElementById("play").disabled="disabled"; document.getElementById("pause").disabled=""; document.getElementById("stop").disabled=""; document.getElementById("slideshow").src=imgsrc[i]; i++; setTimeout("startSlideshow()",1000); } else if(smode=="pause") { document.getElementById("pause").disabled="disabled"; document.getElementById("play").disabled=""; document.getElementById("play").value="Resume"; } else if(smode=="stop") { document.getElementById("play").disabled=""; document.getElementById("play").value="Play"; document.getElementById("pause").disabled="disabled"; document.getElementById("stop").disabled="disabled"; document.getElementById("slideshow").src=imgsrc[0]; i=0; } if(i==imgsrc.length) { i=0; } } </script> </head> <body> <img id="slideshow" src="photos/photo1.jpg" /> <br /> <input id="play" type="button" value="Play" onclick="mode('play');startSlideshow();" /> <input id="pause" type="button" value="Pause" disabled="disabled" onclick="mode('pause');startSlideshow();" /> <input id="stop" type="button" value="Stop" disabled="disabled" onclick="mode('stop');startSlideshow();" /> </body> </html>
See a demo of this Simple Javascript Slideshow. Download the Javascript Slideshow
Great slideshow for us novices.
Can you place a 3 second fade in somehow without to much trouble?
Thank you!
Hey, your codes are great, i appriciate….
thanx alot
Your code is wonderfully simple and clear, unlike so many other slideshow scripts. Is there any chance you could add “Next” and “Previous” arrows, buttons or links?
Thanks,
David