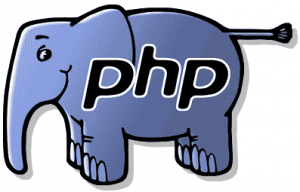
A quick tutorial on how to check for open ports using PHP. This function fsockopen() is used in the Open Port Check Tool in this blog. You can check whether a certain port is open on a specified IP address or hostname. Before reading take note that most FREE web hosting providers disable fsockopen() function as a security measure so first check whether the function can be used. Another thing to take note is that if fsockopen is unable to connect to a port it doesn’t necessarily mean that port is closed on the remote host, your server in which the script is running might also have block the OUTBOUND connection to that port.
Did you land up in this page searching for the Open Port Check Tool ?
Lets go straight to the syntax of fsockopen(). The complete syntax as shown in the official PHP documentation is
resource fsockopen ( string $hostname [, int $port = -1 [, int &$errno [, string &$errstr [, float $timeout = ini_get("default_socket_timeout") ]]]] )
But for this PHP open port check tutorial we’ll use only the first two parameters
fsockopen(hostname,portno)
The fsockopen() function returns a resource identifier if the connection is successful or the Boolean value FALSE if connection couldn’t be established. We’ll use the if else loop to check this out.
<?php if(fsockopen("websistent.com",80)) { print "I can see port 80"; } else { print "I cannot see port 80"; } ?>
Y0u can also replace hostnames with IP addresses in the fsockopen() function. An important thing is that when the script is unable to open a connection to the specified port it may also issue a PHP Warning message “[13-Jul-2011 10:53:09] PHP Warning: fsockopen() [<a href=’function.fsockopen’>function.fsockopen</a>]: unable to connect to hostname:80 (Connection timed out) in /path/to/script.php(42) : eval()’d code on line 20”
To prevent PHP from displaying such errors at runtime read Disabling PHP Display Errors
Hi
Can You share the source for the Open Port check tool ?
Hi Mohammed,
The source is in this article, did you try it?
if I follow the https://websistent.com/disabling-php-display-errors/,
the warning message will gone but it not show my custom error text
(like example in line 8) and stop running to another script too
how to make it show custom error text like you?
Put an ‘@’ before fsockopen –
Hi Jesin.
Very informative articles here!
I do a lot of my own PHP coding too. The “fsockopen()” function is also very useful for checking for some proxies, BTW. Sometimes a specific port is used by a proxy (or even, a “reverse proxy”) and can report as a valid resource (or not, depending on how the server is configured to handle such requests).
Also,
If the “fsockopen” function is disabled (or just not available for that specific system configuration/OS), the CURL library may be of help (again, if it is available and NOT disabled too).
Otherwise,
Once again, a very informative article. 🙂
– Jim
Hello.
Is there a chance to check few ports on locahost like80, 443, 8080 in one request?
if all respond then ok, if 0 then off else something …
Greetings
Hi,
You can try using this condition: